티스토리 뷰
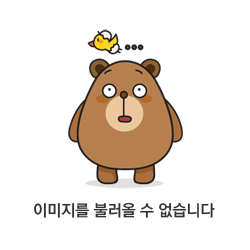
1. 사건의 발단
최근 회사에서 버튼을 누르면 사라졌다 나타는 위젯을 만들어야 할 일이 있었다.
그냥 뭐 간단하게 해결될 일이라고 생각을 했고 처음에는 AnimatedContainer 위젯을 사용해 구현을 하려했다.
Column(
children: [
_header(context),
AnimatedContainer(
height: _height,
duration: const Duration(milliseconds: 300),
child: _child,
),
],
)
대충 위와같이 _header 위젯에 있는 특정 버튼을 누르면 _height 의 크기가 변하면서 자식의 크기가 줄어들거나 늘어나게 만들었었는데 작업 해놓고 보니 간과한것이 있었다..!!!
그것은 바로 AnimatedContainer 의 child 에 들어가는 위젯의 높이가 고정되지 않았다는것,,
flutter는 key를 통해 위젯의 크기를 가져올 수 있지만 위젯이 빌드되기 전까지는 그 크기를 알 방법이 없다. 따라서 _child에 해당하는 위젯의 높이를 사전에 알고있지 않으면 0인상태에서 확장시키는것은 가능하지만 확장을 미리 시켜놓을수는 없는상황,,,, 다른 방법을 찾아야했다 😂 😂 😂
2. ExpansionTile Widget을 사용..?
생각해보니 내가 그려야하는 위젯은 ExpansionTile 의 형태와 매우 유사했다. 동료 직원이 그 점을 짚어주어서 부랴부랴 새로 만들어보았다 ㅎㅎ
ExpansionTile(
title: _header(context),
tilePadding: EdgeInsets.zero,
children: [
_animationChild(),
],
);
그래서 부랴부랴 위와같이 간단하게 만들어봤는데 어라?
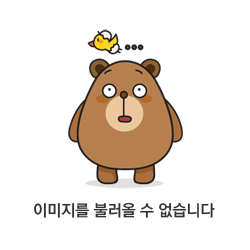
내가 넣은 화살표 말고 오른쪽에 화살표가 기본으로 들어있다.. 그럼 지워야지!!
ExpansionTile(
title: _header(context),
tilePadding: EdgeInsets.zero,
trailing: const SizedBox(),
children: [
_animationChild(),
],
);
trailing <-- 이부분이 저 기본으로 들어가는 화살표 영역인지라 비워두면 저 영역이 아예 사라질줄 알았다.
하지만 그건 내착각이었다
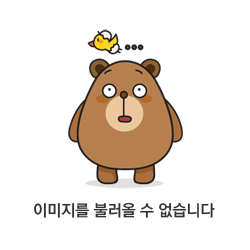
화살표만 사라졌을 뿐 저 영역은 어떻게 해도 사라지지 않았다. 구글링을 해보니 다른사람들도 저 영역을 없애지 못해 커스텀해서 사용을 했다고 한다. 그래 개발이 이렇게 만만할리없지,, 있는거 가져다 쓰려했던 내가 바보다!
3. ExpansionTile을 뜯자!
원하는 결과를 얻지는 못했지만 저 ExpansionTile 이라는 위젯이 자식의 높이를 알지 못해도 확장시켜주는것은 사실이다. 그렇다면 그 비밀은 저 위젯 안에 있을터 나는 ExpansionTile 위젯을 뜯어보기로 했다.
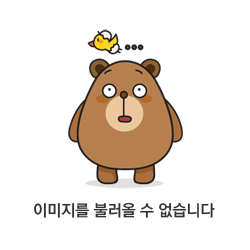
파라미터를 천천히 살펴보면 봐야할 부분이 어딘지 대충 각이 나온다. 'title' 부분이 header 이며, 'children' 부분이 확장되는 영역이렷다!
저 많은 코드들중에 봐야하는 부분은 아랫쪽에 몰려있었다.
class _ExpansionTileState extends State<ExpansionTile> with SingleTickerProviderStateMixin {
static final Animatable<double> _easeOutTween = CurveTween(curve: Curves.easeOut);
static final Animatable<double> _easeInTween = CurveTween(curve: Curves.easeIn);
static final Animatable<double> _halfTween = Tween<double>(begin: 0.0, end: 0.5);
final ColorTween _borderColorTween = ColorTween();
final ColorTween _headerColorTween = ColorTween();
final ColorTween _iconColorTween = ColorTween();
final ColorTween _backgroundColorTween = ColorTween();
late AnimationController _controller;
late Animation<double> _iconTurns;
late Animation<double> _heightFactor;
late Animation<Color?> _borderColor;
late Animation<Color?> _headerColor;
late Animation<Color?> _iconColor;
late Animation<Color?> _backgroundColor;
bool _isExpanded = false;
@override
void initState() {
super.initState();
_controller = AnimationController(duration: _kExpand, vsync: this);
_heightFactor = _controller.drive(_easeInTween);
_iconTurns = _controller.drive(_halfTween.chain(_easeInTween));
_borderColor = _controller.drive(_borderColorTween.chain(_easeOutTween));
_headerColor = _controller.drive(_headerColorTween.chain(_easeInTween));
_iconColor = _controller.drive(_iconColorTween.chain(_easeInTween));
_backgroundColor = _controller.drive(_backgroundColorTween.chain(_easeOutTween));
_isExpanded = PageStorage.of(context)?.readState(context) as bool? ?? widget.initiallyExpanded;
if (_isExpanded)
_controller.value = 1.0;
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
void _handleTap() {
setState(() {
_isExpanded = !_isExpanded;
if (_isExpanded) {
_controller.forward();
} else {
_controller.reverse().then<void>((void value) {
if (!mounted)
return;
setState(() {
// Rebuild without widget.children.
});
});
}
PageStorage.of(context)?.writeState(context, _isExpanded);
});
widget.onExpansionChanged?.call(_isExpanded);
}
// Platform or null affinity defaults to trailing.
ListTileControlAffinity _effectiveAffinity(ListTileControlAffinity? affinity) {
switch (affinity ?? ListTileControlAffinity.trailing) {
case ListTileControlAffinity.leading:
return ListTileControlAffinity.leading;
case ListTileControlAffinity.trailing:
case ListTileControlAffinity.platform:
return ListTileControlAffinity.trailing;
}
}
Widget? _buildIcon(BuildContext context) {
return RotationTransition(
turns: _iconTurns,
child: const Icon(Icons.expand_more),
);
}
Widget? _buildLeadingIcon(BuildContext context) {
if (_effectiveAffinity(widget.controlAffinity) != ListTileControlAffinity.leading)
return null;
return _buildIcon(context);
}
Widget? _buildTrailingIcon(BuildContext context) {
if (_effectiveAffinity(widget.controlAffinity) != ListTileControlAffinity.trailing)
return null;
return _buildIcon(context);
}
Widget _buildChildren(BuildContext context, Widget? child) {
final ExpansionTileThemeData expansionTileTheme = ExpansionTileTheme.of(context);
final Color borderSideColor = _borderColor.value ?? Colors.transparent;
return Container(
decoration: BoxDecoration(
color: _backgroundColor.value ?? expansionTileTheme.backgroundColor ?? Colors.transparent,
border: Border(
top: BorderSide(color: borderSideColor),
bottom: BorderSide(color: borderSideColor),
),
),
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ListTileTheme.merge(
iconColor: _iconColor.value ?? expansionTileTheme.iconColor,
textColor: _headerColor.value,
child: ListTile(
onTap: _handleTap,
contentPadding: widget.tilePadding ?? expansionTileTheme.tilePadding,
leading: widget.leading ?? _buildLeadingIcon(context),
title: widget.title,
subtitle: widget.subtitle,
trailing: widget.trailing ?? _buildTrailingIcon(context),
),
),
ClipRect(
child: Align(
alignment: widget.expandedAlignment
?? expansionTileTheme.expandedAlignment
?? Alignment.center,
heightFactor: _heightFactor.value,
child: child,
),
),
],
),
);
}
@override
void didChangeDependencies() {
final ThemeData theme = Theme.of(context);
final ExpansionTileThemeData expansionTileTheme = ExpansionTileTheme.of(context);
final ColorScheme colorScheme = theme.colorScheme;
_borderColorTween.end = theme.dividerColor;
_headerColorTween
..begin = widget.collapsedTextColor
?? expansionTileTheme.collapsedTextColor
?? theme.textTheme.subtitle1!.color
..end = widget.textColor ?? expansionTileTheme.textColor ?? colorScheme.primary;
_iconColorTween
..begin = widget.collapsedIconColor
?? expansionTileTheme.collapsedIconColor
?? theme.unselectedWidgetColor
..end = widget.iconColor ?? expansionTileTheme.iconColor ?? colorScheme.primary;
_backgroundColorTween
..begin = widget.collapsedBackgroundColor ?? expansionTileTheme.collapsedBackgroundColor
..end = widget.backgroundColor ?? expansionTileTheme.backgroundColor;
super.didChangeDependencies();
}
@override
Widget build(BuildContext context) {
final ExpansionTileThemeData expansionTileTheme = ExpansionTileTheme.of(context);
final bool closed = !_isExpanded && _controller.isDismissed;
final bool shouldRemoveChildren = closed && !widget.maintainState;
final Widget result = Offstage(
offstage: closed,
child: TickerMode(
enabled: !closed,
child: Padding(
padding: widget.childrenPadding ?? expansionTileTheme.childrenPadding ?? EdgeInsets.zero,
child: Column(
crossAxisAlignment: widget.expandedCrossAxisAlignment ?? CrossAxisAlignment.center,
children: widget.children,
),
),
),
);
return AnimatedBuilder(
animation: _controller.view,
builder: _buildChildren,
child: shouldRemoveChildren ? null : result,
);
}
}
생각보다 어렵지는 않은 코드였다. 결국 플러터 해부학 01 편에서 다룬 애니메이션을 약간 응용했을 뿐이다.
위젯을 변경시켜주는 부분은 _heightFactor가 의심이 됐다. 이것은 Animation 객체이고 어떤 애니메이션을 적용할지 정의해놓은 _easyInTween 객체와 함께 _controller에 할당됐다.
즉 _heightFactor 를 부여받은 위젯은 1.0 ~ 0.0 사이를 왔다갔다 하며 줄어들어서 사라졌다가 나타나는 동작을 할것이다.
_isExpanded 변수가 true 일경우 1.0 으로 셋팅해주는것을 보니 확실하다!!
그런데
그럼 이제 이중에 필요한 친구들만 뽑아오는 작업을 거치면 완성이다.
4. ExpasionTile 해부!
나에게 필요한 애니메이션은 딱 하나지만 화살표를 움직여주는 애니메이션을 추가하고 몇가지 주요 위젯들과 함께 가져온 결과는 아래에 있다.
import 'package:flutter/material.dart';
import 'package:flutter_report/feature/main_frame.dart';
import 'package:flutter_report/main.dart';
class ExpansionChildPage extends StatefulWidget {
const ExpansionChildPage({Key? key}) : super(key: key);
@override
State<ExpansionChildPage> createState() => _ExpansionChildPageState();
}
class _ExpansionChildPageState extends State<ExpansionChildPage> with TickerProviderStateMixin {
static final Animatable<double> _easeInTween = CurveTween(curve: Curves.easeIn);
late AnimationController _controller;
late Animation<double> _heightFactor;
late Animation<double> _arrowRotate;
bool _isExpanded = false;
@override
void initState() {
super.initState();
_controller = AnimationController(vsync: this, duration: const Duration(milliseconds: 300));
_heightFactor = _controller.drive(_easeInTween);
_arrowRotate = Tween<double>(begin: 0.0, end: 0.5)
.animate(CurvedAnimation(parent: _controller, curve: Curves.easeInCubic));
if (_isExpanded) {
_controller.value = 1.0;
}
}
@override
Widget build(BuildContext context) {
return MainFrame(
route: FeatureEnum.expansionChild,
child: Column(
children: [
_header(context),
_expandableBody(),
],
),
);
}
Widget _header(BuildContext context){
return Container(
padding: const EdgeInsets.symmetric(horizontal: 16),
width: MediaQuery.of(context).size.width,
height: 60,
decoration: BoxDecoration(
border: Border.all(color: Colors.black12),
),
child: Row(
children: [
const Text('please tab! --->'),
const Spacer(),
GestureDetector(
onTap: () => _handleTap(),
child: RotationTransition(
turns: _arrowRotate,
child: const Icon(Icons.keyboard_arrow_down, size: 20,),
),
),
],
),
);
}
Widget _expandableBody(){
return AnimatedBuilder(
animation: _controller.view,
builder: _buildChild,
child: _animationChild(),
);
}
Widget _buildChild(BuildContext context, Widget? child){
return ClipRect(
child: Align(
alignment: Alignment.center,
heightFactor: _heightFactor.value,
child: child,
),
);
}
Widget _animationChild(){
final bool closed = !_isExpanded && _controller.isDismissed;
return Offstage(
offstage: closed,
child: TickerMode(
enabled: !closed,
child: Container(
width: MediaQuery.of(context).size.width,
height: 200,
color: Colors.brown,
),
),
);
}
void _handleTap() {
setState(() {
_isExpanded = !_isExpanded;
if (_isExpanded) {
_controller.forward();
} else {
_controller.reverse();
}
});
}
}
ClipRect 위젯을 통해 영역을 지정하고 Align 위젯에 heightFactor를 지정해주면 마술처럼 위젯이 줄었다가 늘어난다.
child에 해당하는 영역에 OffStage 라는 위젯이 있는데 이 영역은 Visibilty 위젯처럼 영역은 존재하지만 안보이는게 아닌 영역 자체를 없애버리는 위젯이다.
전체 소스코드는 아래에서 볼 수 있다.
https://github.com/Gyeony95/Flutter-Study/tree/master/flutter_report
GitHub - Gyeony95/Flutter-Study: 플러터 공부용 repo
플러터 공부용 repo. Contribute to Gyeony95/Flutter-Study development by creating an account on GitHub.
github.com
'플러터' 카테고리의 다른 글
[Flutter Flame] Flame 엔진을 사용한 게임 예제 만들어보기 (2) 배경과 자동으로 움직이는 캐릭터 넣기 (1) | 2022.10.08 |
---|---|
[Flutter Flame] Flame 엔진을 사용한 게임 예제 만들어보기 (1) 프로젝트 생성 및 계획 (0) | 2022.09.29 |
[플러터] 플러터 애니메이션을 파헤쳐보자 (0) | 2022.09.10 |
Flutter) Flutter 와 SEO에 관한 이슈 (0) | 2021.07.29 |
Flutter) BLoC 패턴과 Provider패턴 (0) | 2021.03.29 |
- Total
- Today
- Yesterday
- Android
- 플러터
- CHANNELS
- mysql
- github
- redis
- 안드로이드
- Kotlin
- Django
- Hummingbird
- node.js
- Android Studio
- flutter
- django server
- Tutorial
- 해결
- RecyclerView
- password
- Git
- springboot
- 에러해결
- 알고리즘
- socket.io
- 에러
- WAS
- 안드로이드스튜디오
- chatting
- flame
- DART
- 코틀린
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 |